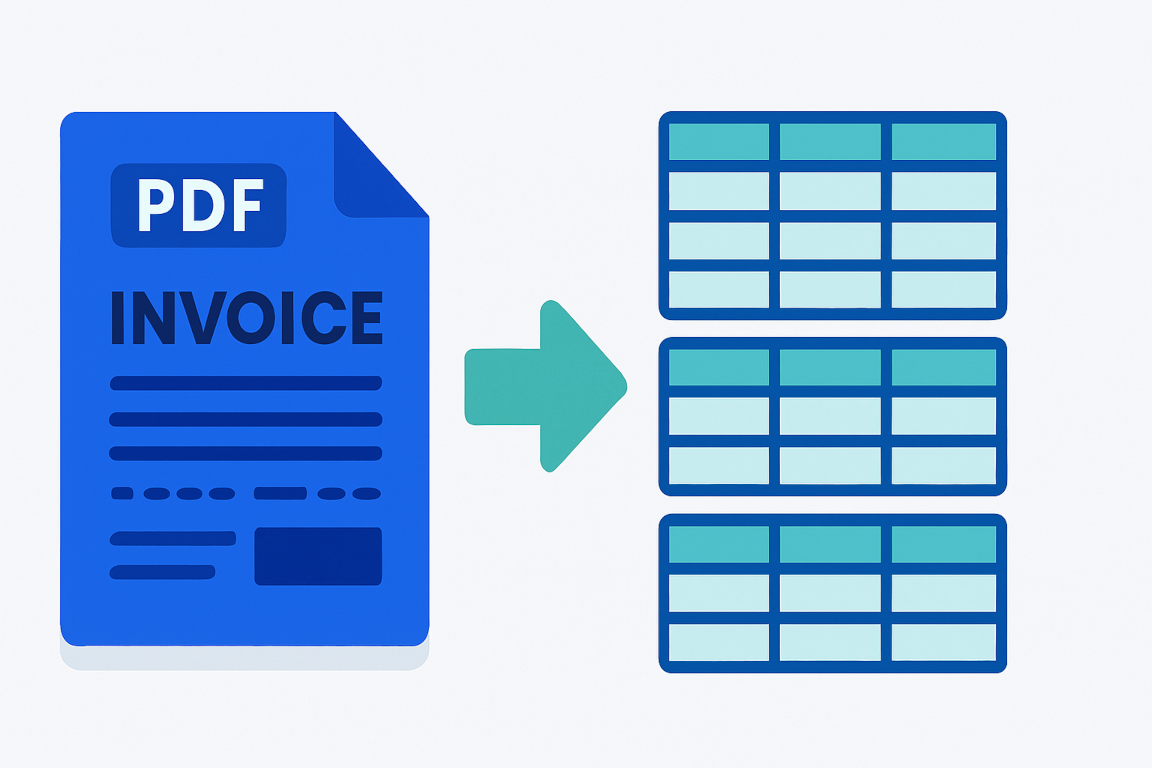
Processing invoices manually can be tedious, time-consuming, and prone to errors. Fortunately, Java provides powerful tools and libraries to automate this repetitive task. Here's how you can create an application to automate invoice processing:
Step 1: Extract Invoice Data from PDFs
Use libraries like Apache PDFBox or Tika to parse PDF invoices and extract relevant data such as invoice number, date, supplier details, and amounts.
PDDocument document = PDDocument.load(new File("invoice.pdf"));
PDFTextStripper stripper = new PDFTextStripper();
String text = stripper.getText(document);
document.close();
// Process text to extract necessary details
Key considerations when extracting PDF data:
- Handle different invoice formats and layouts
- Account for scanned invoices (may require OCR)
- Implement error handling for corrupted files
Step 2: Parse and Structure Data
Use regular expressions, or natural language processing (NLP) libraries such as OpenNLP to accurately identify and structure invoice information.
Pro Tip: Data Extraction Patterns
Create regex patterns for common invoice elements:
- Invoice numbers (INV-2023-001)
- Dates (MM/DD/YYYY or DD-MM-YYYY formats)
- Currency amounts ($1,000.00 or €500,00)
Step 3: Validate and Store Extracted Data
Implement validation logic to verify invoice details. Store validated data into databases like MySQL, PostgreSQL, or MongoDB for persistent storage.
Essential validation checks:
- Cross-check totals with line item sums
- Verify supplier information against your vendor database
- Validate tax calculations
Step 4: Integrate with Accounting Systems
Connect your Java application using REST APIs to accounting platforms like QuickBooks, Xero, or custom-built accounting systems. This allows seamless synchronization of invoice data.
@RestController
public class InvoiceController {
@PostMapping("/api/invoices")
public ResponseEntity<String> uploadInvoice(@RequestBody Invoice invoice) {
// Logic to integrate with accounting API
return ResponseEntity.ok("Invoice processed successfully");
}
}
Step 5: Automate Workflow with Scheduling
Utilize scheduling frameworks such as Quartz Scheduler or Spring's built-in scheduler to run your invoice processing tasks periodically without manual intervention.
Scheduler | Pros | Cons |
---|---|---|
Quartz | Powerful, persistent jobs, clustering | More complex setup |
Spring @Scheduled | Simple, integrated with Spring | Limited features |